Generate random number in Android
To generate random number in Android, class java.util.Random can be used.
This class java.util.Random provides methods that generates pseudo-random numbers of different types, such as int, long, double, and float.
It support two public constructor:
Random() - Construct a random generator with the current time of day in milliseconds as the initial state.
Random(long seed) - Construct a random generator with the given seed as the initial state.
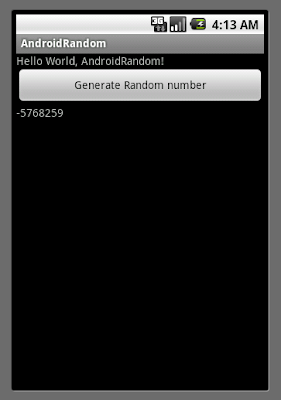
main.xml
AndroidRandom.java
This class java.util.Random provides methods that generates pseudo-random numbers of different types, such as int, long, double, and float.
It support two public constructor:
Random() - Construct a random generator with the current time of day in milliseconds as the initial state.
Random(long seed) - Construct a random generator with the given seed as the initial state.
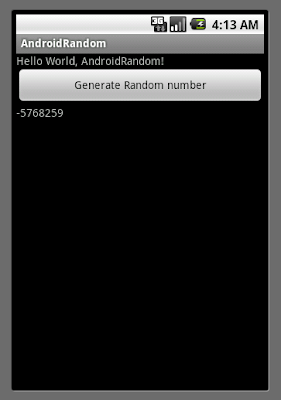
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Generate Random number"
android:id="@+id/generate"
/>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/generatenumber"
/>
</LinearLayout>
AndroidRandom.java
package com.exercise.AndroidRandom;
import java.util.Random;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
public class AndroidRandom extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
final Random myRandom = new Random();
Button buttonGenerate = (Button)findViewById(R.id.generate);
final TextView textGenerateNumber = (TextView)findViewById(R.id.generatenumber);
buttonGenerate.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
textGenerateNumber.setText(String.valueOf(myRandom.nextInt()));
}});
}
}
No comments:
Post a Comment