ProgressBar
ProgressBar is a visual indicator of progress in some operation. Displays a bar to the user representing how far the operation has progressed; the application can change the amount of progress (modifying the length of the bar) as it moves forward.
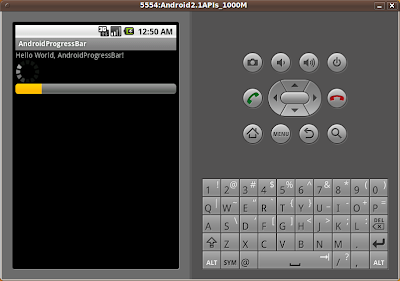
This exercise implemenets a default circle ProgressBar and a Horizontal ProgressBar.
For the Horizontal ProgressBar, a Runnable Thread is implemented to send a message to a Handle to increase the progress of the ProgressBar.
Modify main.xml to add two ProgressBar.
Modify java code.
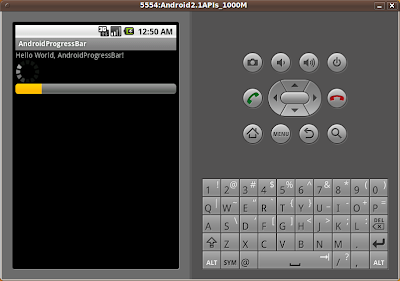
This exercise implemenets a default circle ProgressBar and a Horizontal ProgressBar.
For the Horizontal ProgressBar, a Runnable Thread is implemented to send a message to a Handle to increase the progress of the ProgressBar.
Modify main.xml to add two ProgressBar.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<ProgressBar
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/progressbar_default"
/>
<ProgressBar
android:layout_width="fill_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:id="@+id/progressbar_Horizontal"
android:max="100"
/>
</LinearLayout>
Modify java code.
package com.exercise.AndroidProgressBar;
import android.app.Activity;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.widget.ProgressBar;
public class AndroidProgressBar extends Activity {
ProgressBar myProgressBar;
int myProgress = 0;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
myProgressBar=(ProgressBar)findViewById(R.id.progressbar_Horizontal);
new Thread(myThread).start();
}
private Runnable myThread = new Runnable(){
@Override
public void run() {
// TODO Auto-generated method stub
while (myProgress<100){
try{
myHandle.sendMessage(myHandle.obtainMessage());
Thread.sleep(1000);
}
catch(Throwable t){
}
}
}
Handler myHandle = new Handler(){
@Override
public void handleMessage(Message msg) {
// TODO Auto-generated method stub
myProgress++;
myProgressBar.setProgress(myProgress);
}
};
};
}
No comments:
Post a Comment